How to Set up AWS Lambda with Terraform and Java

Biswambar Pradhan
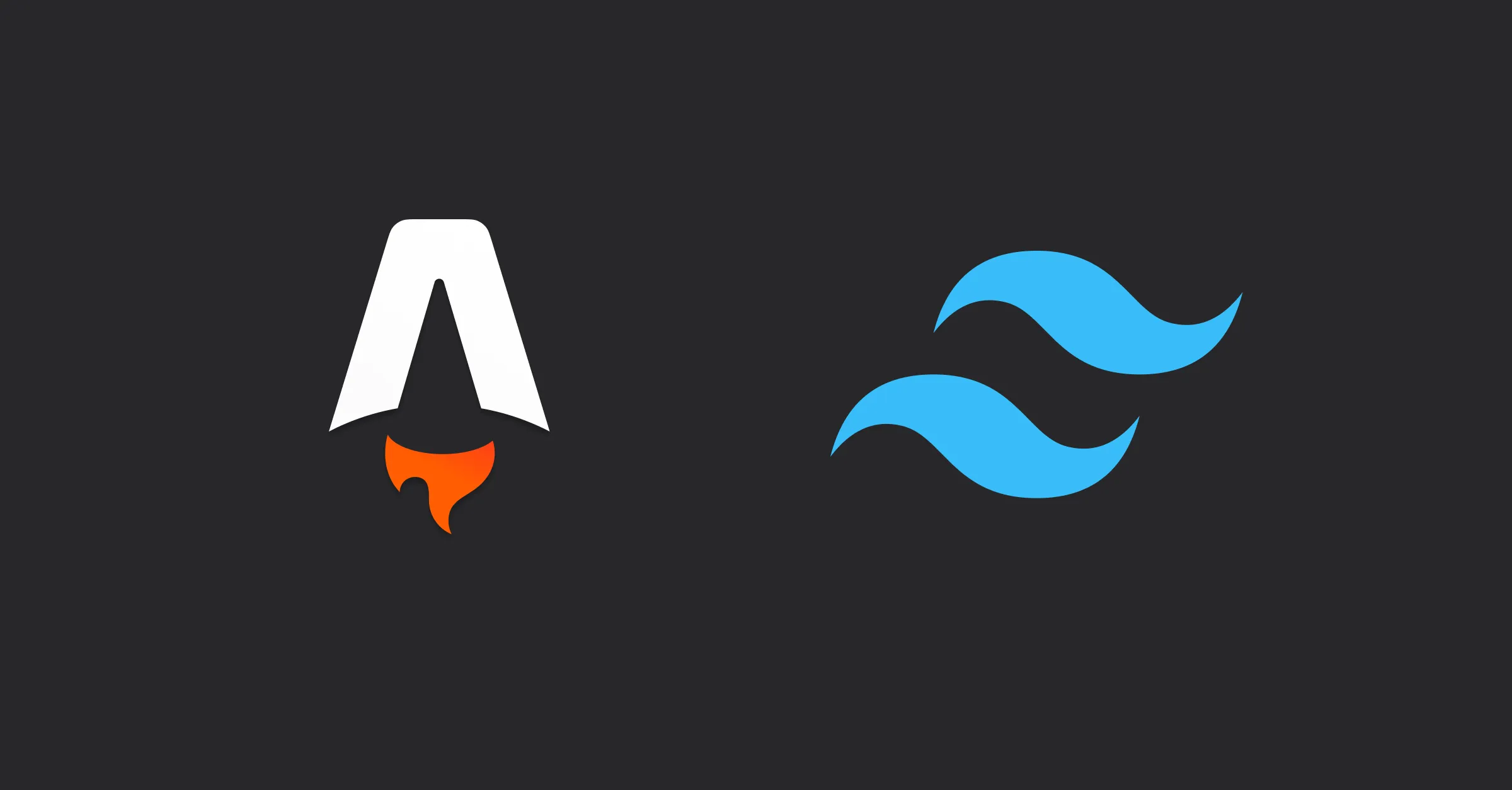
We are going to use 3 files.
- main.tf -> contains the Lambda, provider
- role.tf -> contains IAM role and role permissions
Setup provider with Region
provider "aws" { region = "ap-south-1"}
Create IAM Role
First You need to create an IAM role which Lambda will use while executing the function.
resource "aws_iam_role" "iam_for_lambda" { name = "iam_for_lambda" assume_role_policy = data.aws_iam_policy_document.assume_role.json}
Attach Permissions to the role
data "aws_iam_policy_document" "assume_role" { statement { effect = "Allow"
principals { type = "Service" identifiers = ["lambda.amazonaws.com"] }
actions = ["sts:AssumeRole"] }}
Create Lambda function
resource "aws_lambda_function" "test_lambda" {
# jar file location filename = "java/sample-lambda/target/sample-lambda-1.0-SNAPSHOT.jar" function_name = "sample-lambda" role = aws_iam_role.iam_for_lambda.arn
/* Provide the handler class location in package
for example your Handler.java class in inside abc.xyz lambda handler should be abc.xyz.Handler */ handler = "in.learnaws.Handler"
runtime = "java11"
environment { variables = { foo1 = "bar1", foo2 = "bar2" } }}
Initiate terraform and configure aws cli
terrform initInitializing the backend...
Initializing provider plugins...- Finding latest version of hashicorp/aws...- Finding latest version of hashicorp/archive...- Installing hashicorp/archive v2.4.2...- Installed hashicorp/archive v2.4.2 (signed by HashiCorp)- Installing hashicorp/aws v5.35.0...- Installed hashicorp/aws v5.35.0 (signed by HashiCorp)
Configure AWS Cli
aws configure## provide you access keys and region
AWS Access Key ID [****************UIDX]:AWS Secret Access Key [****************PYia]:Default region name [ap-south-1]:Default output format [json]:
Create java code for Lambda
You can setup java code in separte folder also and provide the jar file path in “filename” Genere the jar using maven command
sample code link java code for Lambda
maven package
[INFO] ------------------------------------------------------------------------[INFO] BUILD SUCCESS[INFO] ------------------------------------------------------------------------[INFO] Total time: 0.937 s[INFO] Finished at: 2024-02-04T12:47:45+05:30
Run Terraform Apply
You can run terraform plan to verify the changes.
terraform apply
Terraform will perform the following actions:
# aws_iam_role.iam_for_lambda will be created + resource "aws_iam_role" "iam_for_lambda" { + arn = (known after apply) + assume_role_policy = jsonencode( { + Statement = [ + { + Action = "sts:AssumeRole" + Effect = "Allow" + Principal = { + Service = "lambda.amazonaws.com" } }, ] + Version = "2012-10-17" } ) + create_date = (known after apply) + force_detach_policies = false + id = (known after apply) + managed_policy_arns = (known after apply) + max_session_duration = 3600 + name = "iam_for_lambda" + name_prefix = (known after apply) + path = "/" + tags_all = (known after apply) + unique_id = (known after apply) }
# aws_lambda_function.test_lambda will be created + resource "aws_lambda_function" "test_lambda" { + architectures = (known after apply) + arn = (known after apply) + filename = "java/sample-lambda/target/sample-lambda-1.0-SNAPSHOT.jar" + function_name = "sample-lambda" + handler = "in.learnaws.Handler" + id = (known after apply) + invoke_arn = (known after apply) + last_modified = (known after apply) + memory_size = 128 + package_type = "Zip" + publish = false + qualified_arn = (known after apply) + qualified_invoke_arn = (known after apply) + reserved_concurrent_executions = -1 + role = (known after apply) + runtime = "java11" + signing_job_arn = (known after apply) + signing_profile_version_arn = (known after apply) + skip_destroy = false + source_code_hash = (known after apply) + source_code_size = (known after apply) + tags_all = (known after apply) + timeout = 3 + version = (known after apply)
+ environment { + variables = { + "foo1" = "bar1" + "foo2" = "bar2" } } }
Plan: 2 to add, 0 to change, 0 to destroy.
Do you want to perform these actions? Terraform will perform the actions described above. Only 'yes' will be accepted to approve.
Enter a value: yes